Detecting malicious payloads across multiple packets v0.2
As you can expect, we are currently working hard for the next release which will introduce new interesting features: protocol dissection, pattern matching, API improvements, etc.
In the initial Haka version, we leveraged on Lua built-in engine to match network data against malicious patterns. The major drawback of this pattern matching engine is that it is not enough expressive (e.g. alternation | is not supported) and does not support partial matching.
Next release will feature a new stream-based regular expression engine based on PCRE. This module allows us to detect accurately a malicious payload scattered over multiple packets:
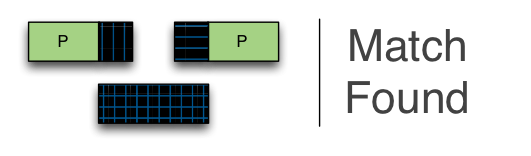
In the following example, we load the regular expression module and compile the malicious pattern which represents a classic /bin/sh shellcode. The second parameter of compile function is an option allowing us to ignore white space chars and comments.
As you have noticed, the syntax of security rules has slightly changed. Rules are event-based. In our example, the security rule is meant to be evaluated whenever a new data is available on the established connection. However, with the additional option streamed, evaluation is done only once waiting for new data to proceed.
The rest of the rule is relative to attack reaction. In our case, we simply raise an alert.
require('protocol/ipv4')
require('protocol/tcp')
local tcp_connection = require('protocol/tcp_connection')
local rem = require('regexp/pcre')
local shellcode = "%xeb %x1f %x5e %x89 %x76 %x08 %x31 %xc0" ..
"%x88 %x46 %x07 %x89 %x46 %x0c %xb0 %x0b" ..
"%x89 %xf3 %x8d %x4e %x08 %x8d %x56 %x0c" ..
"%xcd %x80 %x31 %xdb %x89 %xd8 %x40 %xcd" ..
"%x80 %xe8 %xdc %xff %xff %xff /bin/sh"
local re = rem.re:compile(shellcode, rem.re.EXTENDED)
haka.rule {
hook = tcp_connection.events.receive_data,
options = {
streamed = true,
},
eval = function (flow, iter)
-- match malicious pattern across multiple packets
local ret = re:match(iter)
if ret then
haka.alert{
description = "/bin/sh shellcode detected",
targets = haka.alert.address(flow.dstip)
}
end
end
}
Besides the partial matching capabilities, the new regular expression engine has significantly improved text-based protocol dissection.